基于 Android android-14.0.0_r28 的源代码,来分析 Handler 消息处理机制在 Java 层的实现逻辑
源码路径
framework/base/core/java/andorid/os/ |
---|
| Handler.java
Looper.java
Message.java
MessageQueue.java
HandlerThread.java
HandlerExecutor.java
|
Android 里的四大组件的启动和运行 ( Activity/Service/ContentProvider/Broadcast ) 都是通过 Handler 的消息通信机制来驱动的.
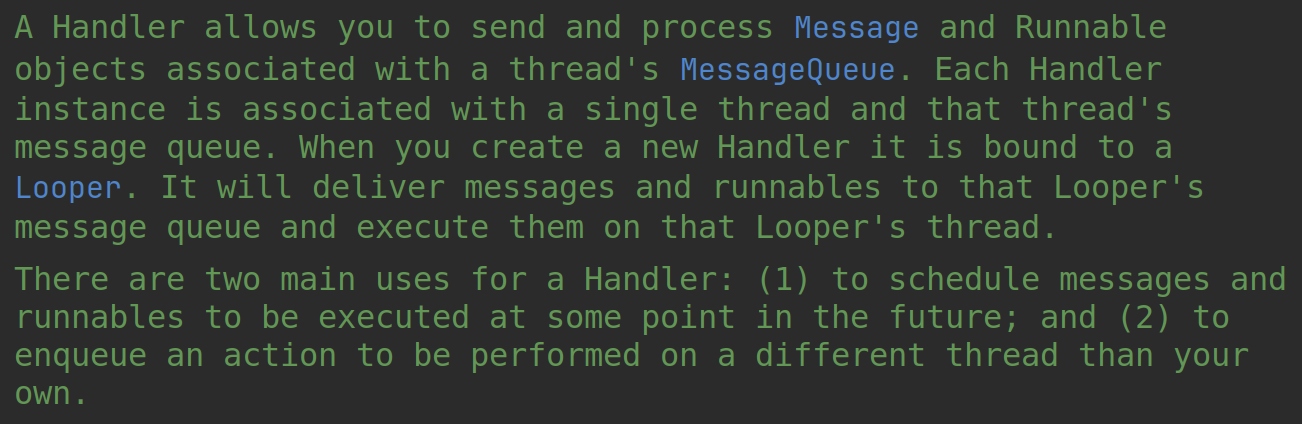
- Message:消息对象,分为硬件产生的消息(如按钮、触摸)和软件生成的消息;
- MessageQueue:消息队列,主要功能是消息入队(MessageQueue.enqueueMessage)和消息获取(MessageQueue.next);
- Handler:消息辅助类,主要功能是发送各种消息事件(Handler.sendMessage)和处理相应消息事件(Handler.handleMessage);
- Looper:整个 Handler 消息机制的发动机. 通过不停的循环(Looper.loop),按机制将消息取出并分发给目标处理者(Handler)处理。
- HandlerThread: 使用辅助类, 方便在子线程创建 Handler
- HandlerExecutor: Executor的包装类, 在源码中被标记了 hide. 实际上 context.getMainExecutor 获取到的就是 HandlerExecutor 对象
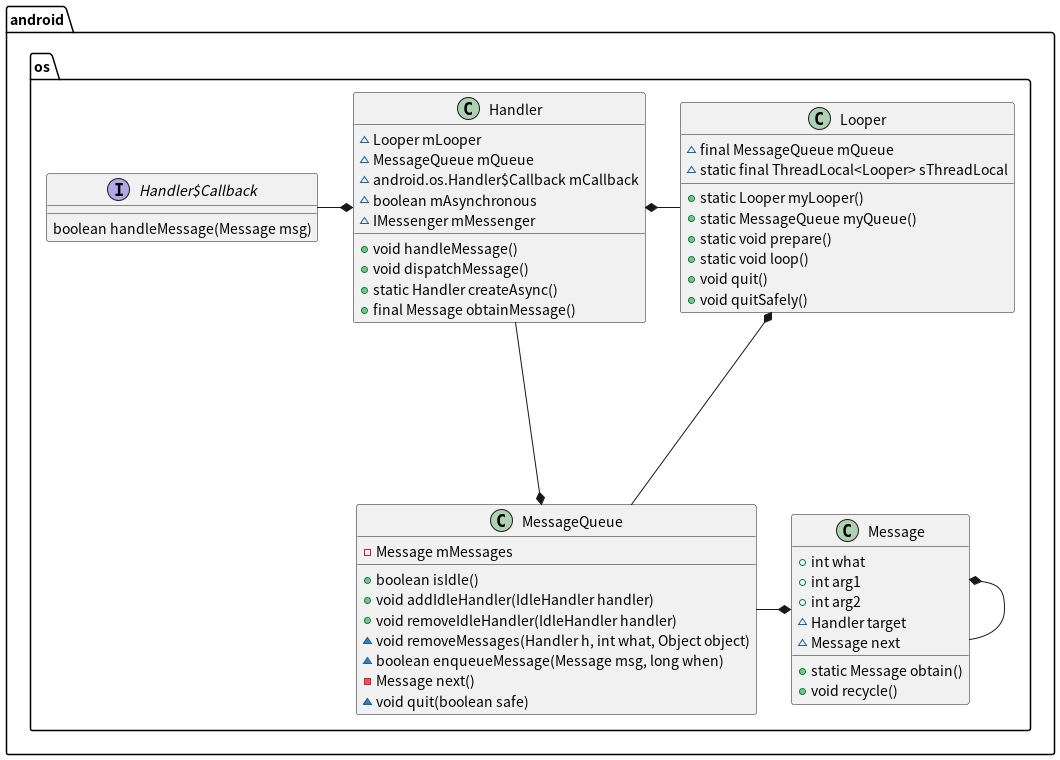
无参方法调用了私有方法 Looper.prepare(boolean quitAllowed)
默认传递参数是 true
, 就是说所有由开发者创建的 Looper 都必须是可退出的. 只有在 prepareMainLooper()
中创建 sMainLooper
时传递的参数为 false
.
framework/base/core/java/andorid/os/Looper.java |
---|
| private static void prepare(boolean quitAllowed) {
if (sThreadLocal.get() != null) {
throw new RuntimeException("Only one Looper may be created per thread");
}
sThreadLocal.set(new Looper(quitAllowed));
}
public static @Nullable Looper myLooper() {
return sThreadLocal.get();
}
|
从源码可以看到Looper.prepare()
在每个线程内只允许执行一次,负责创建 Looper 对象并保存到当前线程的 sThreadLocal
中。
Looper 的构造方法内会创建一个 MessageQueue 对象并获取到当前所在线程对象.
framework/base/core/java/andorid/os/Looper.java |
---|
| private Looper(boolean quitAllowed) {
mQueue = new MessageQueue(quitAllowed); // 创建消息队列
mThread = Thread.currentThread();
}
|
ThreadLocal
用于提供线程局部变量。每个线程都有自己的本地存储区域,不同线程之间无法互相访问彼此的本地存储区域。
ThreadLocal 是没有链表的类 HashMap 结构,数据模型:

ThreadLocal.set
方法, 将 value 存储在本地存储区域.
| public void set(T value) {
Thread t = Thread.currentThread();
ThreadLocalMap map = getMap(t);
if (map != null)
map.set(this, value);
else
createMap(t, value);
}
|
ThreadLocal.get
方法, 将 value 从本地存储区域取出.
| public T get() {
Thread t = Thread.currentThread();
ThreadLocalMap map = getMap(t);
if (map != null) {
ThreadLocalMap.Entry e = map.getEntry(this);
if (e != null) {
@SuppressWarnings("unchecked")
T result = (T)e.value;
return result;
}
}
return setInitialValue();
}
|
framework/base/core/java/andorid/os/Looper.java |
---|
| public static void loop() {
final Looper me = myLooper();
if (me == null) {
throw new RuntimeException("No Looper; Looper.prepare() wasn't called on this thread.");
}
if (me.mInLoop) {
//如果多次执行 loop(), 会在当前消息完成之前执行队列内的其他消息.
//简单理解就是会造成消息执行顺序和入队顺序不一致,消息执行顺序类似并发的状态
//实际上 ThreadLocal 的实现方案保证了每个线程绑定的 Looper 对象只会被 loop 一次
Slog.w(TAG, "Loop again would have the queued messages be executed"
+ " before this one completed.");
}
me.mInLoop = true;
// 确保在权限检查时基于本地进程。
// Make sure the identity of this thread is that of the local process,
// and keep track of what that identity token actually is.
Binder.clearCallingIdentity();
final long ident = Binder.clearCallingIdentity();
// 允许通过 adb 命令修改线程策略
// Allow overriding a threshold with a system prop. e.g.
// adb shell 'setprop log.looper.1000.main.slow 1 && stop && start'
final int thresholdOverride =
SystemProperties.getInt("log.looper."
+ Process.myUid() + "."
+ Thread.currentThread().getName()
+ ".slow", 0);
me.mSlowDeliveryDetected = false;
for (;;) {
if (!loopOnce(me, ident, thresholdOverride)) {
return;
}
}
}
|
原来的消息执行相关代码单独抽成了 loopOnce 方法,整体逻辑没有大的变动
framework/base/core/java/andorid/os/Looper.java |
---|
| private static boolean loopOnce(final Looper me,
final long ident, final int thresholdOverride) {
// 获取需要执行的消息
Message msg = me.mQueue.next(); // might block
if (msg == null) {
// No message indicates that the message queue is quitting.
return false;
}
// 消息执行开始的 log 信息, 消息执行结束也有对应的 log 信息.
// logging 默认是 null 可以通过 setMessageLogging 设置
// 这一对 log 信息各个 Android 版本都没啥变化,很多监控库的实现里面都依赖这个
// This must be in a local variable, in case a UI event sets the logger
final Printer logging = me.mLogging;
if (logging != null) {
logging.println(">>>>> Dispatching to " + msg.target + " "
+ msg.callback + ": " + msg.what);
}
// 新版新增了 Observer 用于回调 message 的而执行状态
// 默认是 LooperStats 对象,很遗憾 setObserver 被标记为了 hide
// Make sure the observer won't change while processing a transaction.
final Observer observer = sObserver;
final long traceTag = me.mTraceTag;
// 设置晚交付消息/慢调度消息的阀值以及状态
// 阀值是可以通过 adb 修改的,可以用来测试一些消息调度/执行的问题
long slowDispatchThresholdMs = me.mSlowDispatchThresholdMs;
long slowDeliveryThresholdMs = me.mSlowDeliveryThresholdMs;
if (thresholdOverride > 0) {
slowDispatchThresholdMs = thresholdOverride;
slowDeliveryThresholdMs = thresholdOverride;
}
final boolean logSlowDelivery = (slowDeliveryThresholdMs > 0) && (msg.when > 0);
final boolean logSlowDispatch = (slowDispatchThresholdMs > 0);
final boolean needStartTime = logSlowDelivery || logSlowDispatch;
final boolean needEndTime = logSlowDispatch;
if (traceTag != 0 && Trace.isTagEnabled(traceTag)) {
Trace.traceBegin(traceTag, msg.target.getTraceName(msg));
}
final long dispatchStart = needStartTime ? SystemClock.uptimeMillis() : 0;
final long dispatchEnd;
// token 由 messageDispatchStarting 生成, 并且在后续的结束/异常状态回调中作为参数传递.主要用于标识一个消息的完成,所以原则上应该是唯一的
Object token = null;
if (observer != null) {
token = observer.messageDispatchStarting();
}
long origWorkSource = ThreadLocalWorkSource.setUid(msg.workSourceUid);
try {
// 消息真正的开始执行
msg.target.dispatchMessage(msg);
// 消息执行完毕回调
if (observer != null) {
observer.messageDispatched(token, msg);
}
dispatchEnd = needEndTime ? SystemClock.uptimeMillis() : 0;
} catch (Exception exception) {
// 消息执行异常回调
if (observer != null) {
observer.dispatchingThrewException(token, msg, exception);
}
throw exception;
} finally {
ThreadLocalWorkSource.restore(origWorkSource);
if (traceTag != 0) {
Trace.traceEnd(traceTag);
}
}
// 打印晚交付消息日志,队列执行完成之前只会打印一次
if (logSlowDelivery) {
if (me.mSlowDeliveryDetected) {
if ((dispatchStart - msg.when) <= 10) {
Slog.w(TAG, "Drained");
me.mSlowDeliveryDetected = false;
}
} else {
if (showSlowLog(slowDeliveryThresholdMs, msg.when, dispatchStart, "delivery",
msg)) {
// Once we write a slow delivery log, suppress until the queue drains.
me.mSlowDeliveryDetected = true;
}
}
}
// 打印慢消息日志
if (logSlowDispatch) {
showSlowLog(slowDispatchThresholdMs, dispatchStart, dispatchEnd, "dispatch", msg);
}
// 消息执行结束的 log 信息, 相比于 observer 机制晚很多且不知到执行结果
if (logging != null) {
logging.println("<<<<< Finished to " + msg.target + " " + msg.callback);
}
// Make sure that during the course of dispatching the
// identity of the thread wasn't corrupted.
final long newIdent = Binder.clearCallingIdentity();
if (ident != newIdent) {
Log.wtf(TAG, "Thread identity changed from 0x"
+ Long.toHexString(ident) + " to 0x"
+ Long.toHexString(newIdent) + " while dispatching to "
+ msg.target.getClass().getName() + " "
+ msg.callback + " what=" + msg.what);
}
msg.recycleUnchecked();
return true;
}
|
quit 实际调用的是 MessageQueue 的 quit 方法. 调用 quit 之后无法再向队列发送消息.
- 当 safe = true 时,只移除尚未触发的所有消息(message.when > now),对于正在触发的消息并不移除;
- 当 safe = flase 时,移除所有的消息
framework/base/core/java/andorid/os/Looper.java |
---|
| public void quit() {
mQueue.quit(false);
}
public void quitSafely() {
mQueue.quit(true);
}
|
默认使用当前线程TLS中的Looper对象,并且 callback 为 null,消息为同步处理方式。
framework/base/core/java/andorid/os/Handler.java |
---|
| @Deprecated
public Handler() {
this(null, false);
}
@Deprecated
public Handler(@Nullable Callback callback) {
this(callback, false);
}
//hide
public Handler(@Nullable Callback callback, boolean async) {
//匿名类、内部类或本地类都必须申明为static,否则会警告可能出现内存泄露
if (FIND_POTENTIAL_LEAKS) {
final Class<? extends Handler> klass = getClass();
if ((klass.isAnonymousClass() || klass.isMemberClass() || klass.isLocalClass()) &&
(klass.getModifiers() & Modifier.STATIC) == 0) {
Log.w(TAG, "The following Handler class should be static or leaks might occur: " +
klass.getCanonicalName());
}
}
mLooper = Looper.myLooper();
// 当前线程要前置执行过 Looper.prepare() 方法, 否则会抛出异常。
if (mLooper == null) {
throw new RuntimeException(
"Can't create handler inside thread " + Thread.currentThread()
+ " that has not called Looper.prepare()");
}
mQueue = mLooper.mQueue;
mCallback = callback;
mAsynchronous = async;
}
|
需要指定 Looper,默认 callback 为 null 且消息为同步处理方式。
framework/base/core/java/andorid/os/Handler.java |
---|
| public Handler(@NonNull Looper looper) {
this(looper, null, false);
}
public Handler(@NonNull Looper looper, @Nullable Callback callback) {
this(looper, callback, false);
}
// hide
@UnsupportedAppUsage
public Handler(@NonNull Looper looper, @Nullable Callback callback, boolean async) {
mLooper = looper;
mQueue = looper.mQueue;
mCallback = callback;
mAsynchronous = async;
}
|
需要指定 Looper,默认 callback 为 null 且消息为异步处理方式。
framework/base/core/java/andorid/os/Handler.java |
---|
| @NonNull
public static Handler createAsync(@NonNull Looper looper) {
if (looper == null) throw new NullPointerException("looper must not be null");
return new Handler(looper, null, true);
}
|
从方法调用示意图可以看到,消息发送最终收敛到了 MessageQueue.enqueueMessage()
.
区别只是不同的方法对消息的构造参数不同.
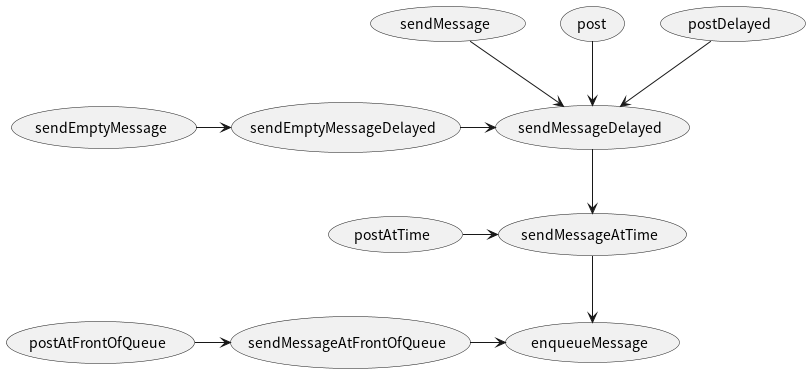
framework/base/core/java/andorid/os/Handler.java |
---|
| private boolean enqueueMessage(@NonNull MessageQueue queue, @NonNull Message msg,
long uptimeMillis) {
msg.target = this;
msg.workSourceUid = ThreadLocalWorkSource.getUid();
if (mAsynchronous) {
msg.setAsynchronous(true);
}
return queue.enqueueMessage(msg, uptimeMillis);
}
|
在 Looper.loopOnce()
中, 提到过消息的执行msg.target.dispatchMessage(msg);
framework/base/core/java/andorid/os/Handler.java |
---|
| public void dispatchMessage(@NonNull Message msg) {
// Message 的 callback 不为空.直接在当前线程执行 msg.callback.run() 方法
// 主要是以 Runnable 为参数的几个 post 方法发送的 message 会走到这
if (msg.callback != null) {
handleCallback(msg);
} else {
// Handler 的 mCallback 不为空. 调用 mCallback.handleMessage() 方法
// mCallback 可以让开发者在非必要的场景避免创建 Handler 子类
if (mCallback != null) {
if (mCallback.handleMessage(msg)) {
return;
}
}
//Handler 自身的方法 handleMessage()
handleMessage(msg);
}
}
|
framework/base/core/java/andorid/os/Handler.java |
---|
| public final Message obtainMessage()
{
return Message.obtain(this);
}
|
framework/base/core/java/andorid/os/Handler.java |
---|
| public final void removeCallbacks(@NonNull Runnable r) {
mQueue.removeMessages(this, r, null);
}
public final void removeMessages(int what) {
mQueue.removeMessages(this, what, null);
}
|
MessageQueue 是消息机制的核心,作为 Java 层和 C++ 层的连接纽带,大部分核心方法都交给 native 层来处理:
framework/base/core/java/andorid/os/MessageQueue.java |
---|
| private native static long nativeInit();
private native static void nativeDestroy(long ptr);
@UnsupportedAppUsage
private native void nativePollOnce(long ptr, int timeoutMillis); /*non-static for callbacks*/
private native static void nativeWake(long ptr);
private native static boolean nativeIsPolling(long ptr);
private native static void nativeSetFileDescriptorEvents(long ptr, int fd, int events);
|
先按照上文顺序,把 Java 层涉及到 MessageQueue 的方法介绍下.
framework/base/core/java/andorid/os/MessageQueue.java |
---|
| Message next() {
// Return here if the message loop has already quit and been disposed.
// This can happen if the application tries to restart a looper after quit
// which is not supported.
// 检查 Native 层的指针,如果为 0 则表示已经退出了,并且重启无效
// 这个指针是在 MessageQueue 创建时候通过 nativeInit 获取的
final long ptr = mPtr;
if (ptr == 0) {
return null;
}
int pendingIdleHandlerCount = -1; // -1 only during first iteration
int nextPollTimeoutMillis = 0;
for (;;) {
if (nextPollTimeoutMillis != 0) {
Binder.flushPendingCommands();
}
//阻塞操作,当等待超时(nextPollTimeoutMillis)或者消息队列被唤醒的时候返回
//
nativePollOnce(ptr, nextPollTimeoutMillis);
synchronized (this) {
// Try to retrieve the next message. Return if found.
final long now = SystemClock.uptimeMillis();
Message prevMsg = null;
Message msg = mMessages;
// barrier message 的特征: 没有用来执行它的 Handler 对象
// 这个逻辑保证了 barrier 会阻塞住所有的同步消息
if (msg != null && msg.target == null) {
// Stalled by a barrier. Find the next asynchronous message in the queue.
do {
prevMsg = msg;
msg = msg.next;
} while (msg != null && !msg.isAsynchronous());
}
if (msg != null) {
if (now < msg.when) {
// 还没到下一个消息的执行时间,设置超时时间,等待被唤醒
// Next message is not ready. Set a timeout to wake up when it is ready.
nextPollTimeoutMillis = (int) Math.min(msg.when - now, Integer.MAX_VALUE);
} else {
// 找到可执行的消息
// Got a message.
mBlocked = false;
if (prevMsg != null) {
prevMsg.next = msg.next;
} else {
mMessages = msg.next;
}
msg.next = null;
if (DEBUG) Log.v(TAG, "Returning message: " + msg);
// 标识使用中并返回
msg.markInUse();
return msg;
}
} else {
// No more messages.
nextPollTimeoutMillis = -1;
}
// 处于退出状态,已经处理完消息队列并返回 null
// Process the quit message now that all pending messages have been handled.
if (mQuitting) {
dispose();
return null;
}
// 前面找不到可执行的消息,说明这段时间空闲了,接下来处理 IdleHandler
// 如果消息队列时空的或者第一个消息还不可以执行的时候,看下有多少 IdleHandler
// 注意:配合代码 117 行, pendingIdleHandlerCount < 0 保证了每次 next() 期间只有一次机会执行 IdleHandler
// If first time idle, then get the number of idlers to run.
// Idle handles only run if the queue is empty or if the first message
// in the queue (possibly a barrier) is due to be handled in the future.
if (pendingIdleHandlerCount < 0
&& (mMessages == null || now < mMessages.when)) {
pendingIdleHandlerCount = mIdleHandlers.size();
}
// 没有 IdleHandler 可以执行,继续等待
if (pendingIdleHandlerCount <= 0) {
// No idle handlers to run. Loop and wait some more.
// 没有消息处理,也没有 IdleHandler,说明本次循环彻底闲下来了
mBlocked = true;
continue;
}
if (mPendingIdleHandlers == null) {
mPendingIdleHandlers = new IdleHandler[Math.max(pendingIdleHandlerCount, 4)];
}
mPendingIdleHandlers = mIdleHandlers.toArray(mPendingIdleHandlers);
}
// 利用空闲时间执行 IdleHandler
// Run the idle handlers.
// We only ever reach this code block during the first iteration.
for (int i = 0; i < pendingIdleHandlerCount; i++) {
final IdleHandler idler = mPendingIdleHandlers[i];
mPendingIdleHandlers[i] = null; // release the reference to the handler
boolean keep = false;
try {
keep = idler.queueIdle();
} catch (Throwable t) {
Log.wtf(TAG, "IdleHandler threw exception", t);
}
// 返回 fase 表示这个 IdleHandler 只需要执行一次
// 返回 true 表示这个 IdleHandler 需要多次执行
if (!keep) {
synchronized (this) {
mIdleHandlers.remove(idler);
}
}
}
// 注意: 呼应代码 75 行,pendingIdleHandlerCount = 0 保证了每次 next() 期间只有一次机会执行 IdleHandler
// Reset the idle handler count to 0 so we do not run them again.
pendingIdleHandlerCount = 0;
// 回头检查下是不是有新的消息不需要等待就可以执行了
// While calling an idle handler, a new message could have been delivered
// so go back and look again for a pending message without waiting.
nextPollTimeoutMillis = 0;
}
}
|
MessageQueue 是按照 Message 触发时间的顺序排列, enqueueMessage 会遍历队列并将新消息插入到合适的位置
framework/base/core/java/andorid/os/MessageQueue.java |
---|
| boolean enqueueMessage(Message msg, long when) {
// 消息必须有对应的 Handler 来处理
if (msg.target == null) {
throw new IllegalArgumentException("Message must have a target.");
}
synchronized (this) {
// 消息还在使用中
if (msg.isInUse()) {
throw new IllegalStateException(msg + " This message is already in use.");
}
// 即将退出了
if (mQuitting) {
IllegalStateException e = new IllegalStateException(
msg.target + " sending message to a Handler on a dead thread");
Log.w(TAG, e.getMessage(), e);
msg.recycle();
return false;
}
// 消息对象设置各个状态和属性
msg.markInUse();
msg.when = when;
Message p = mMessages;
boolean needWake;
// 消息插入到队头
if (p == null || when == 0 || when < p.when) {
// New head, wake up the event queue if blocked.
msg.next = p;
mMessages = msg;
needWake = mBlocked;
} else {
// 消息插入到队中间
// Inserted within the middle of the queue. Usually we don't have to wake
// up the event queue unless there is a barrier at the head of the queue
// and the message is the earliest asynchronous message in the queue.
needWake = mBlocked && p.target == null && msg.isAsynchronous();
Message prev;
for (;;) {
prev = p;
p = p.next;
if (p == null || when < p.when) {
break;
}
if (needWake && p.isAsynchronous()) {
needWake = false;
}
}
msg.next = p; // invariant: p == prev.next
prev.next = msg;
}
// 需要唤醒的情形:
// 当前循环彻底闲着没事干的时候, 1. 有新消息插在了队列头部; 2. 队列头是个 barrier 而且当前消息是最早的异步消息
// We can assume mPtr != 0 because mQuitting is false.
if (needWake) {
nativeWake(mPtr);
}
}
return true;
}
|
采用了两个 while 循环, 先砍头后剔骨的方式移除掉全部符合条件的消息
framework/base/core/java/andorid/os/MessageQueue.java |
---|
| void removeMessages(Handler h, int what, Object object) {
if (h == null) {
return;
}
synchronized (this) {
Message p = mMessages;
// 先砍头,把符合条件的消息都移除掉
// Remove all messages at front.
while (p != null && p.target == h && p.what == what
&& (object == null || p.obj == object)) {
Message n = p.next;
mMessages = n;
p.recycleUnchecked();
p = n;
}
// 后剔骨,把不再头部但符合条件的消息都移除掉
// Remove all messages after front.
while (p != null) {
Message n = p.next;
if (n != null) {
if (n.target == h && n.what == what
&& (object == null || n.obj == object)) {
Message nn = n.next;
n.recycleUnchecked();
p.next = nn;
continue;
}
}
p = n;
}
}
}
|
Barriers are indicated by messages with a null target whose arg1 field carries the token.
- postSyncBarrier 不会唤醒 Looper
- removeSyncBarrier 可能会唤醒 Looper
framework/base/core/java/andorid/os/MessageQueue.java |
---|
| private int postSyncBarrier(long when) {
// Enqueue a new sync barrier token.
// We don't need to wake the queue because the purpose of a barrier is to stall it.
synchronized (this) {
final int token = mNextBarrierToken++;
final Message msg = Message.obtain();
msg.markInUse();
msg.when = when;
msg.arg1 = token;
Message prev = null;
Message p = mMessages;
if (when != 0) {
while (p != null && p.when <= when) {
prev = p;
p = p.next;
}
}
if (prev != null) { // invariant: p == prev.next
msg.next = p;
prev.next = msg;
} else {
msg.next = p;
mMessages = msg;
}
return token;
}
}
public void removeSyncBarrier(int token) {
// Remove a sync barrier token from the queue.
// If the queue is no longer stalled by a barrier then wake it.
synchronized (this) {
Message prev = null;
Message p = mMessages;
while (p != null && (p.target != null || p.arg1 != token)) {
prev = p;
p = p.next;
}
if (p == null) {
throw new IllegalStateException("The specified message queue synchronization "
+ " barrier token has not been posted or has already been removed.");
}
final boolean needWake;
if (prev != null) {
prev.next = p.next;
needWake = false;
} else {
mMessages = p.next;
needWake = mMessages == null || mMessages.target != null;
}
p.recycleUnchecked();
// If the loop is quitting then it is already awake.
// We can assume mPtr != 0 when mQuitting is false.
if (needWake && !mQuitting) {
nativeWake(mPtr);
}
}
}
|
Message 作为整个消息机制的调度对象,主要包含以下内容:
数据类型 |
成员变量 |
解释 |
int |
what |
消息类别 |
long |
when |
消息触发时间 |
int |
arg1 |
参数1 |
int |
arg2 |
参数2 |
Bundle |
data |
扩展参数 |
Object |
obj |
消息内容 |
Handler |
target |
消息响应方 |
Runnable |
callback |
回调方法 |
从缓存池中取出一个对象,并清理 flags
framework/base/core/java/andorid/os/Message.java |
---|
| public static Message obtain() {
synchronized (sPoolSync) {
if (sPool != null) {
Message m = sPool;
sPool = m.next;
m.next = null;
m.flags = 0; // clear in-use flag
sPoolSize--;
return m;
}
}
return new Message();
}
|
清理 Message 对象的参数并放入缓存池,但是这里遗留了 FLAG_IN_USE
, 会在 obtain
时清除
framework/base/core/java/andorid/os/Message.java |
---|
| public void recycle() {
if (isInUse()) {
if (gCheckRecycle) {
throw new IllegalStateException("This message cannot be recycled because it "
+ "is still in use.");
}
return;
}
recycleUnchecked();
}
void recycleUnchecked() {
// Mark the message as in use while it remains in the recycled object pool.
// Clear out all other details.
flags = FLAG_IN_USE;
what = 0;
arg1 = 0;
arg2 = 0;
obj = null;
replyTo = null;
sendingUid = UID_NONE;
workSourceUid = UID_NONE;
when = 0;
target = null;
callback = null;
data = null;
synchronized (sPoolSync) {
if (sPoolSize < MAX_POOL_SIZE) { //当消息池没有满时(50个),将 Message 对象加入消息池
next = sPool;
sPool = this;
sPoolSize++;
}
}
}
|